In the mobile app development community, the term “design patterns” often surfaces in discussions among developers, designers, and tech enthusiasts.
But what exactly are design patterns, and why are they crucial in mobile development? In this blog, we’ll delve into the significance of understanding design patterns in the mobile development landscape.
What Are Design Patterns?
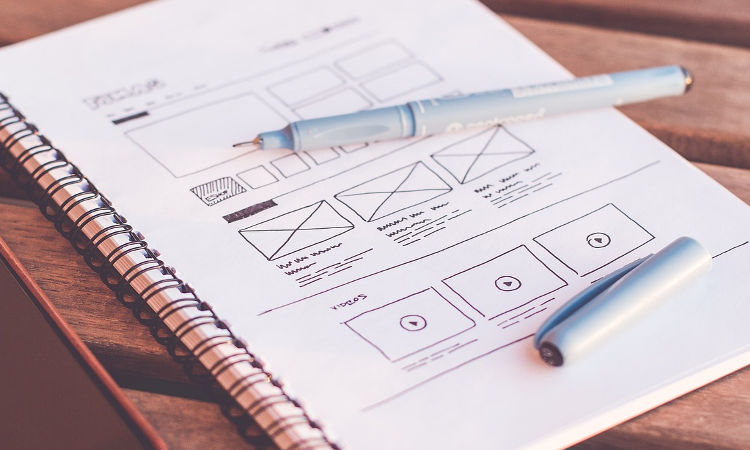
To put it simply, design patterns are proven solutions to recurring design problems. They aren’t necessarily lines of code but rather templates or blueprints for solving common issues encountered during the development process. Design patterns contain the best, proven practices, offering developers a structured approach to building scalable, maintainable, and efficient software.
BM Insight: BrightMarbles Group is best known for its ability to build software solutions from scratch. But we do more than just guide clients from ideation through coding to product launch; as illustrated by our CXO Nevena Nemeš in her article Empowering Brands through Full-Spectrum Digital Services: BrightMarbles Approach, we offer full-fledged marketing and design services to our loyal clients.
The Matter of Relevance in Mobile Development
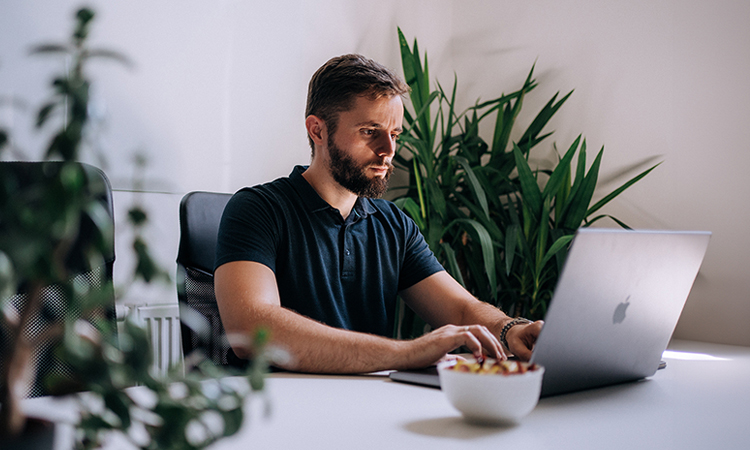
Mobile applications come with their own set of challenges, ranging from varying screen sizes and resolutions to limited device resources. Design patterns serve as invaluable tools in overcoming these challenges and streamlining the development process. Here’s why they matter:
- Enhancing Code Maintainability. One of the primary benefits of design patterns is their ability to enhance code maintainability. By adhering to established patterns like MVC (Model-ViewController) or MVVM (Model-View-ViewModel), developers can organize their codebase in a clear and logical manner. This makes it easier to understand, debug, and modify the code, both for the original developers and those who may work on it in the future.
- Promoting Code Reusability. Design patterns encourage the reuse of code components, leading to more efficient development cycles. Mobile apps often require similar functionalities across different screens or modules. By employing patterns like Singleton or Factory, developers can create reusable components that can be leveraged throughout the application, minimizing redundancy and speeding up development.
- Facilitating Scalability. As mobile apps grow in complexity, scalability becomes a crucial aspect of development. Design patterns offer scalability by providing flexible architectures that can accommodate future changes and additions without requiring a complete overhaul of the codebase. Patterns like Observer or Decorator enable developers to design modular and extensible systems, allowing for seamless integration of new features.
- Improving Performance Efficiency. This is a paramount feature in mobile development, where resources such as battery life and network bandwidth are limited. Design patterns help optimize performance by promoting efficient algorithms and data structures. Patterns like Flyweight or Proxy can reduce memory footprint and network overhead, resulting in faster and more responsive applications.
- Fostering Collaboration. In collaborative development environments, where multiple developers may be working on the same project simultaneously, design patterns serve as a common language. They provide a standardized way of approaching design problems, making it easier for developers to communicate and understand each other’s code.
Recommended Design Patterns
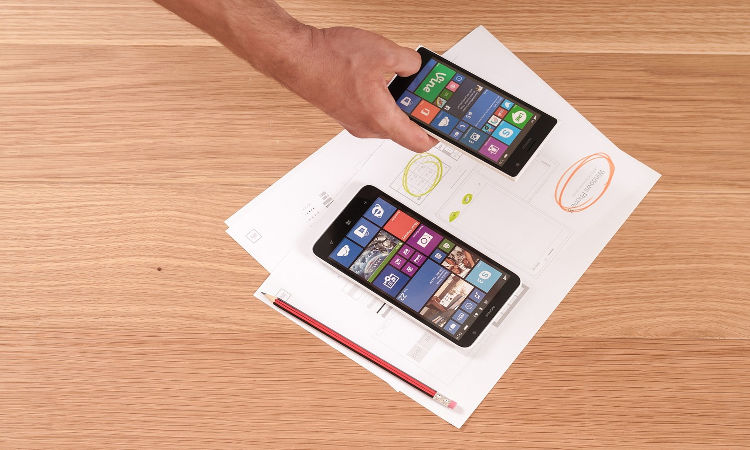
Design Patterns that you should focus on first (I’ll try to explain them in plain examples):
Observer
Imagine you have a favorite YouTube channel, and you want to know whenever they upload a new video. So, you hit the notification bell. Whenever they post a video, YouTube notifies you, and you can watch it. In this scenario: You are the observer. The YouTube channel is the subject. The notification bell is like the subscription mechanism that connects you (the observer) to the YouTube channel (the subject).
Whenever a new video is uploaded (state change), YouTube sends you a notification (update), so you know there’s something new to watch. So, the Observer pattern is like subscribing to notifications from your favorite channel, so you’re always aware of updates without having to constantly check.
Decorator
Let’s say you have a plain cupcake, and you want to make it more exciting by adding frosting, sprinkles, and maybe even a cherry on top. Each decoration adds something extra to the cupcake without changing its basic cupcake-ness. In programming: The plain cupcake is like your original object. The frosting, sprinkles, and cherry are decorators.
Each decorator adds extra functionality or behavior to the original object. You can combine decorators in different ways to create new combinations of functionality, just like you can combine frosting, sprinkles, and cherries to create different kinds of cupcakes. So, the Decorator pattern is like adding toppings to a cupcake, allowing you to enhance or modify an object’s behavior dynamically without changing its core structure.
Factory
Imagine you’re at a pizza place, and you want to order a pizza. Instead of telling the chef how to make the pizza from scratch every time, you just tell the waiter what type of pizza you want (like pepperoni or vegetarian). The waiter then goes to the kitchen, tells the chef what you want, and the chef makes the pizza for you.
In programming: You are the client who wants a pizza. The waiter is like the factory, which takes your order and decides which type of pizza to create. The chef is like the Factory method, responsible for creating the specific type of pizza you ordered. That’s why the Factory pattern is like ordering a pizza at a restaurant: you tell the factory what you want, and it gives you the right kind of object without you having to worry about how it’s made.
Singleton
The Singleton pattern is like having a magic cookie jar in your kitchen. No matter how many times someone opens the pantry, there’s only ever one cookie jar. If someone wants a cookie, they go to the same jar every time, ensuring that everyone gets cookies from the same source.
In programming, the Singleton pattern ensures that there’s only one instance of a class, no matter how many times you try to create it. It’s like always getting cookies from the same jar, maintaining consistency across your code.
Some other patterns that you should check out about are:
- Command
- Adapter
- Facade
- Template
- Method
- Iterator
- Compose
- State
- Proxy
There are more but if you are just starting try focusing on this list first.
Design Patterns and Reference Books
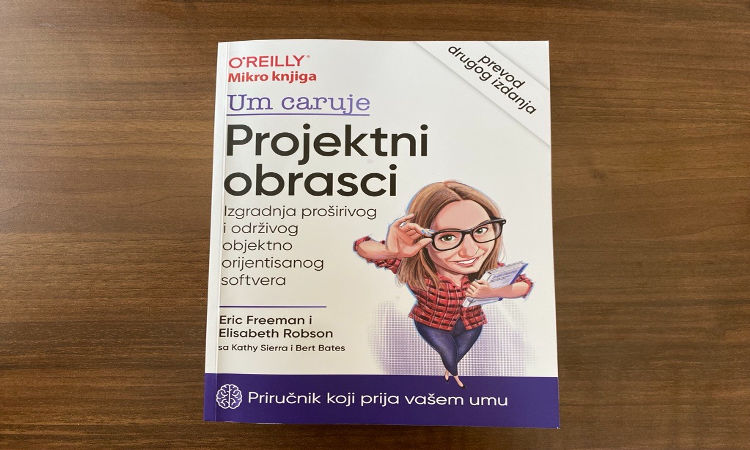
“Head First Design Patterns: A Brain-Friendly Guide” and “Design Patterns: Elements of Reusable Object-Oriented Software” (often referred to as the “Gang of Four” book) are two well-known resources in software engineering and design patterns. Here’s a brief overview and comparison of the two:
- Approach and Style:
“Head First Design Patterns”: This book, part of the “Head First” series, employs a highly visual and engaging style of teaching. It uses illustrations, diagrams, anecdotes, and hands-on exercises to explain design patterns. The content is structured in a way that aims to be easy to understand and retain.
- Design Patterns:
The “Gang of Four” book takes a more formal and academic approach. It dives deep into the theory behind design patterns, providing detailed explanations, UML diagrams, and code examples. It’s often considered denser and more technical compared to “Head First Design Patterns.” - Target Audience:
“Head First Design Patterns”: This book is often recommended for beginners or those who are new to design patterns and objectoriented design. Its conversational tone and interactive approach make it accessible to a wide range of readers, including self-taught programmers. - Design Patterns:
GoF: The “Gang of Four” book is better suited for readers with some background in software development and design. It’s widely regarded as a seminal work in the field and is often recommended for intermediate to advanced developers who want a deeper understanding of design patterns. - Content:
Both books cover the same set of design patterns originally cataloged by the Gang of Four (GoF), including creational, structural, and behavioral patterns. “Head First Design Patterns” emphasizes practical application and problem-solving. It focuses on how to use patterns effectively in real-world scenarios, with an emphasis on practical implementation and best practices.
“Design Patterns: Elements of Reusable Object-Oriented Software” provides more comprehensive explanations of the design patterns, discussing their motivations, applicability, variations, and potential consequences. It also includes discussions on software engineering principles and best practices. - Language and Examples:
“Head First Design Patterns” uses Java as its primary language for code examples. The book focuses more on the concepts rather than specific programming languages, making it adaptable to developers familiar with other languages.
“Design Patterns: Elements of Reusable Object-Oriented Software” primarily features code examples in C++ and Smalltalk, reflecting the languages prevalent at the time of its writing. However, the principles discussed are applicable to a wide range of object-oriented languages.
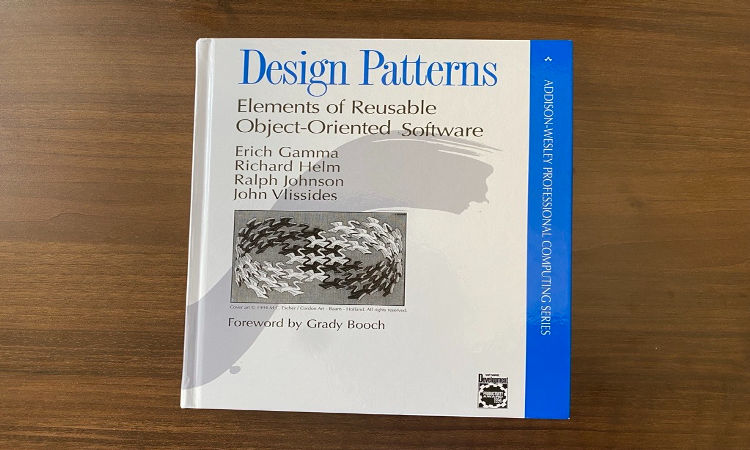
While both “Head First Design Patterns” and “Design Patterns: Elements of Reusable Object-Oriented Software” cover the same core set of design patterns, they differ in their approach, style, and target audience.
“Head First Design Patterns” is more accessible and practical, suitable for beginners, while “Design Patterns: Elements of Reusable Object-Oriented Software” is more comprehensive and theoretical, geared towards intermediate to advanced developers.
Conclusion
No matter where you decide to start learning about Design Patterns, one thing is certain: it’s essential to begin. Embracing these fundamental concepts will undoubtedly elevate your skills and proficiency in software development, empowering you to tackle complex problems with confidence and creativity.
About Author
Marko Krstanovic is a software engineer and technical officer with a proven track record of delivering exceptional mobile applications. His expertise spans various programming languages and technologies, including iOS and Flutter, which he has used to create seamless and secure client apps. With over five years of experience, Marko is a true innovator in the tech industry, constantly pushing the boundaries of what’s possible in mobile app development.