If we could choose one perfect metaphor to describe the journey to creating and maintaining clean, elegant code, we would say it is akin to conducting a symphony.
Martin Fowler’s “Refactoring: Improving the Design of Existing Code” is the score that guides developers through this intricate musical landscape.
In this piece, we will embark on an immersive exploration of this masterpiece, as we delve into the essence of refactoring. We will also decipher the nuanced language of code smells, wield the tools from the refactoring catalog, and dance through the intricate steps of test-driven refactoring.
Prepare to be enlightened by the harmonious blend of theory and practical examples that shape the art of code evolution.
The Essence of Refactoring

At the heart of “Refactoring” is the profound concept of code evolution. Unlike the conventional wisdom that views code as a static entity, Fowler advocates the idea that code is a living, breathing creation that must adapt to change.
The essence of refactoring lies in the ability to make small, consistent improvements to the code without altering its external behavior. As we unravel this fundamental concept, let’s analyze a real-world example.
BM Insight: As a trailblazer in applying cutting-edge softdev methodologies, BrightMarbles Group Holding embraces the principles of code evolution and every new proven concept that improves final software products.
Simplifying Complex Logic
Consider a method with convoluted conditional logic:
public double calculateTotalPrice(Order order) {
double totalPrice = 0.0;
// Complex logic determining price based on order
details
if (order.isPromoCodeApplied()) {
totalPrice = applyPromoCode(order);
} else {
totalPrice = calculateRegularPrice(order);
}
return totalPrice;
}
This code smells of conditional complexity difficult to understand and maintain. Applying the refactoring technique “Replace Conditional with Polymorphism” could transform this into a more elegant structure:
public double calculateTotalPrice(Order order) {
return order.calculatePrice();
}
By employing polymorphism and moving the pricing logic into the Order class, we’ve simplified the method, making it more readable and maintainable.
The Code Smells: Recognizing Opportunities for Change
Fowler introduces the concept of “code smells“– the subtle indicators that suggest areas in the code that may benefit from refactoring. Let’s explore a few common code smells and understand how they guide us toward opportunities for improvement.
Duplicated Code
Duplicated code is a clear indication that a refactoring opportunity exists. Consider the following example:
public class OperationExample {
public int performOperationA(int x, int y) {
// Common logic for both operations
int intermediateResult = x + y;
int finalResult = intermediateResult * 3;
// Additional logic specific to operation A...
return finalResult;
}
public int performOperationB(int x, int y) {
// Common logic for both operations
int intermediateResult = x + y;
int finalResult = intermediateResult * 3;
// Additional logic specific to operation B...
return finalResult;
}
// Other methods in the class...
}
This code smells of duplication, and applying the “Extract Method” refactoring technique can eliminate redundancy:
public class OperationExample {
public int performOperationA(int x, int y) {
int result = performCommonOperation(x, y);
// Additional logic specific to operation A...
return result;
}
public int performOperationB(int x, int y) {
int result = performCommonOperation(x, y);
// Additional logic specific to operation B...
return result;
}
private int performCommonOperation(int x, int y) {
int intermediateResult = x + y;
int finalResult = intermediateResult * 3;
return finalResult;
}
// Other methods in the class...
}
By extracting the common logic into a separate method, we’ve eliminated duplication, enhancing readability and maintainability.
Long Method
A method that’s excessively long another code smell. Consider:
public void processOrder(Order order) {
// Lengthy logic A
// ...
// Lengthy logic B
// ...
// Lengthy logic C
// ...
}
This method is challenging to understand and modify. Applying the “Extract Method” refactoring can break it down into smaller, easily manageable pieces:
public void processOrder(Order order) {
processLogicA(order);
processLogicB(order);
processLogicC(order);
}
private void processLogicA(Order order) {
// Logic A
// ...
}
private void processLogicB(Order order) {
// Logic B
// ...
}
private void processLogicC(Order order) {
// Logic C
// ...
}
By dividing the long method into smaller, focused methods, we’ve improved code organization and readability.
The Refactoring Catalog: A Toolbox for Improvement
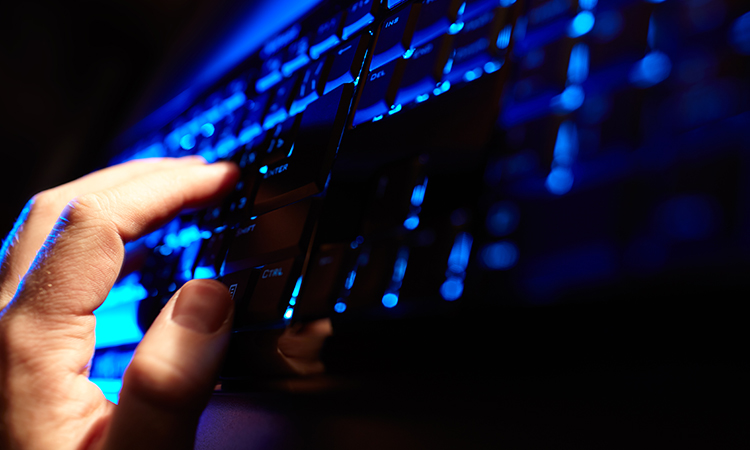
Fowler’s refactoring catalog is a rich toolbox of techniques to address specific code issues. Let’s explore a few key refactorings and their applications:
Extract Method
The Extract Method refactoring allows us to break down complex code into smaller, easy-to-handle methods.
Example:
public double calculateTotalPrice(Order order) {
double totalPrice = calculateBasePrice(order);
totalPrice += calculateTax(order);
totalPrice += calculateShippingCost(order);
return totalPrice;
}
private double calculateBasePrice(Order order) {
// Base price calculation logic
// ...
}
private double calculateTax(Order order) {
// Tax calculation logic
// ...
}
private double calculateShippingCost(Order order) {
// Shipping cost calculation logic
// ...
}
By extracting each calculation into its own method, we enhance code modularity, which is now easier to understand and maintain.
Rename Method
The Rename Method refactoring is a simple yet powerful technique for improving code readability.
Example:
public double calcTotalPrice(Order order) {
// Calculation logic
// ...
}
This method name is unclear. Applying the Rename Method refactoring can clarify its purpose:
public double calculateTotalPrice(Order order) {
// Calculation logic
// ...
}
By giving the method a clear and descriptive name, we improve code readability and convey its purpose more effectively.
Test-Driven Refactoring: A Symphony of Confidence
Fowler underscores the importance of a test-driven approach to refactoring. Tests act as guardians, so that each refactoring step maintains the correctness of the code. Let’s explore a practical example of test-driven refactoring.
Example: Refactoring with Tests
Consider a method for calculating the factorial of a number:
public int calculateFactorial(int n) {
if (n == 0) {
return 1;
} else {
return n * calculateFactorial(n - 1);
}
}
To ensure the accuracy of this method, we can write a set of tests:
@Test
public void testFactorialOfZero() {
assertEquals(1, calculateFactorial(0));
}
@Test
public void testFactorialOfFive() {
assertEquals(120, calculateFactorial(5));
}
With the tests in place, we can confidently refactor the code, applying the “Replace Recursion with Iteration” technique:
public int calculateFactorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
Such tests ensure that the refactoring maintains the expected behavior of the code. This test-driven approach provides a safety net, allowing developers to make changes with confidence.
The Human Element: Navigating the Challenges of Refactoring
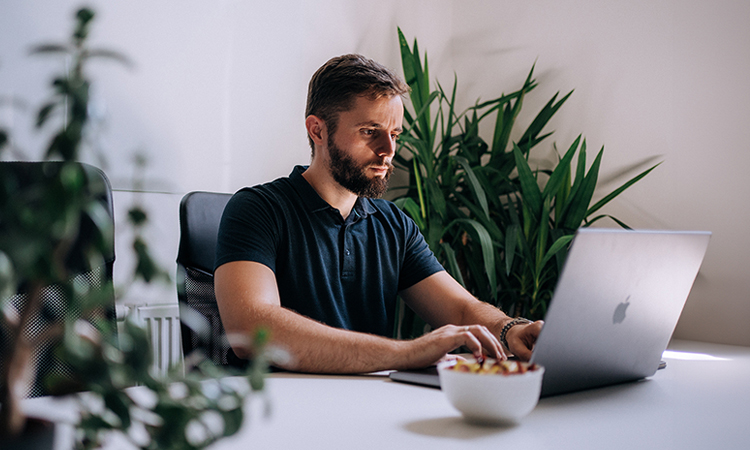
The technical aspects of refactoring are crucial, but Fowler also acknowledges the human side of the equation.
Convincing stakeholders, estimating time for refactoring, and dealing with legacy code are real challenges. Let’s explore how these challenges can be successfully resolved.
Convincing Stakeholders
To convince non-technical stakeholders of the value of refactoring, create a compelling narrative that highlights the business benefits of refactoring. Those may vary, from improved maintainability and reduced bug count to faster feature delivery.
Estimating Refactoring Time
Accurately estimating the time required for refactoring can be complex. Hence, break down refactoring tasks into smaller, more manageable units, and use historical data to inform your estimates. Communicate the benefits of refactoring, with an emphasis on long-term gains.
Dealing with Legacy Code
Legacy code often poses challenges for refactoring. Therefore, prioritize areas of the codebase that are critical for business functionality or prone to bugs.
Gradually introduce refactoring into your development workflow, balancing it with new feature development.
A Paradigm Shift in Mindset
Reading “Refactoring” has ignited a paradigm shift in my mindset as a developer. I now view code as a dynamic entity that evolves over time, rather than an immutable artifact.
The pursuit of clean, maintainable code is no longer a destination but a continuous journey. As we internalize this shift, let’s go back to a poignant quote from the book:
“Any fool can write code that a computer can understand. Good programmers write code that humans can understand.” – Martin Fowler
Closing Thoughts: A Must-Read for Every Developer
In conclusion, “Refactoring” by Martin Fowler stands as a timeless guide for developers at all stages of their careers. It is a manifesto for the art of crafting code that not only functions but also serves as a point of inspiration for developers who cherish it.
As I apply the principles learned from this book in my day-to-day coding tasks, I find myself not just writing code but orchestrating a symphony of elegance and efficiency. May this exploration into the world of refactoring inspire you to embark on your own journey of code evolution, creating software that resonates with the beauty of well-crafted music.
About Author
Marko Krstanovic is a software engineer and technical officer with a proven track record of delivering exceptional mobile applications. His expertise spans various programming languages and technologies, including iOS and Flutter, which he has used to create seamless and secure client apps. With over five years of experience, Marko is a true innovator in the tech industry, constantly pushing the boundaries of what’s possible in mobile app development.