Accidental coding errors can be a huge headache for developers, as even the most experienced coders can make mistakes that can prevent the code from functioning properly. Such errors can cause a lot of time and money to be wasted on debugging.
Fortunately, a variety of tools are available to help prevent these errors from occurring in JavaScript projects, including the popular ESLint, Prettier, and Husky.
3 of the Most Popular JS Tools for Preventing Errors
ESLint
The best way to prevent accidental coding errors is to use a linter, and the most widely used one for JavaScript is ESLint.
It’s a handy tool that thoroughly scans your code and gives you feedback on any possible errors, so you can fix them before they lead to any issues.
Prettier
Prettier is an invaluable code formatting tool that helps make your code more readable and consistent.
By automatically reformatting your code according to a set of rules, it ensures that all of your code follows the same style, making for a cleaner and more efficient coding experience.
Husky
Husky is an incredibly useful tool that can help to prevent careless coding mistakes by running checks on your code before it is committed.
It can run linting programs like ESLint and formatting programs like Prettier to make sure that the code meets your standards.
This allows developers to catch any errors before they are pushed to the codebase, making life a lot easier for the whole team.
Setting Up ESLint, Prettier, and Husky
Setting up ESLint, Prettier, and Husky is relatively easy.
To get started, you’ll need to install the following packages:
// Run these commands in the project root
$ npm install eslint prettier husky --save-dev
If you are using yarn, $ yarn add eslint prettier husky –dev
Next, you’ll need to configure the packages.
// ESLint
Create an .eslintrc file in the project root:
{
"env": {
"browser": true,
"node": true
},
"rules": {
"semi": ["error", "always"],
"quotes": ["error", "double"]
}
}
// Prettier
Create a .prettierrc file in the project root:
{
"semi": true,
"singleQuote": true
}
// Husky
Add the following to your package.json file:
"husky":
{
"hooks": {
"pre-commit": "eslint && prettier"
}
}
With these configuration files in place, ESLint, Prettier, and Husky will run automatically when code is committed and pushed.
Benefits of Using Prettier, Husky, and ESLint to Increase the Code Security of Your Project
Debugging problems that could have been avoided with the linter can be really time-consuming. Moreover, it can be difficult to analyze Git changes due to different IDE formatting preferences among team members – changes that do not affect the app’s functionality, but are merely code formatting.
By utilizing some of the powerful JavaScript debugging tools, you can not only reduce the time spent on these issues, but also ensure that the code is consistent and professional-looking, which makes development a much more pleasant experience.
How We Leveraged the 3 Most Popular Debugging Tools: Linting Against Staged GIT Files (lint-staged)
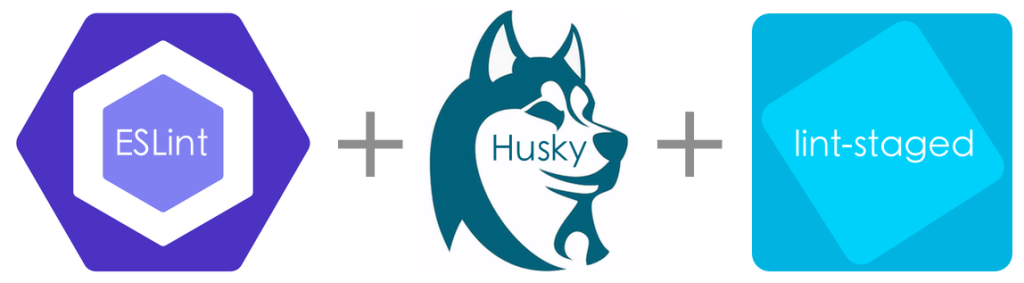
Lint-staged is a Git hook combined with Husky, which we used together with ESLint and Prettier for formatting. This package runs before the actual commit and applies the rules that we defined.
Therefore, even if a file is mistakenly saved with incorrect formatting, this hook will still run and override the formatting with the format we’ve specified.
Lint-staged can be installed by running the following command in the terminal:
npm install --save-dev lint-staged
If you are using yarn, yarn add lint-staged –dev
Additionally, we’ve defined which folders to ignore, as these folders contain models auto-generated by the backend.
“lint-staged”:
{
"*.{js,tsx,ts, tsx}": "eslint --max-warnings 0 --cache --fix --cache-location './node_modules/@eslint/.eslintcache/'",
"!(src/api/**/*).{js,jsx, scss,css,md,ts,tsx}": "prettier --write"
}
Bonus
We hope that this article has been useful in helping you protect your project against accidental errors by utilizing ESLint, Prettier, and Husky. Doing so should save you much trouble in the future, and make your project more organized and efficient.
By gaining an in-depth understanding of the ESLint tool and why it identifies certain constructs as warnings or errors, you can not only increase your knowledge of JavaScript, but also learn some valuable rules to apply when developing projects in this language. To further strengthen your knowledge on this topic, here are some helpful resources:
- Using ESLint with TypeScript
- All the rules supported by ESLint
- Suggestion to add the integration described in this article to the CRA
- Basic ESLint configuration in the Create React App
- Linting messages in commits
- The origins of Prettier
- ESLint –fix flag
- Pages of the tools used here, such as Husky, Prettier, and ESLint