Hi, frontend devs and preferably other JS enthusiasts’,
Please grab yourself a cup of coffee, relax and go through this lightweight list of cool tips and tricks every FE developer should know or at least be aware of at some point in their career.
1. Simple Logging With console.log()
Here is a simple one to start. Every FE dev uses a console to debug their code, right? You know those times when you want to console.log() a simple value and check what you get in browser dev tools? It turns out you could utilize the power of ES6 and mix it up with a bit of logic and log stuff to the console like.
const myVariable = “simple string”;
const anotherVariable = [1, 2, “random”];
const thirdOne = {someProp: ‘value’};
console.log({myVariable, anotherVariable, thirdOne})
This is actually a shorthand of writing
console.log({myVariable: myVariable, anotherVariable: anotherVariable, thirdOne: thirdOne})
Which lets you easily track which value belongs to which variable assignment. Sure, this hardly makes sense for simple logging, but if you want to log multiple values to the console, it could come in handy.
2. Debugging Without console.log()
And for those who dislike using console.log() in your code, try spinning up your dev build in the browser, firing up the dev tools, and selecting the sources tab. Afterward, you can CMD + P / CTRL + P and choose the desired file you’d like to debug (like normalize.ts in the example below) and debug the line when the code interpreter tackles it.
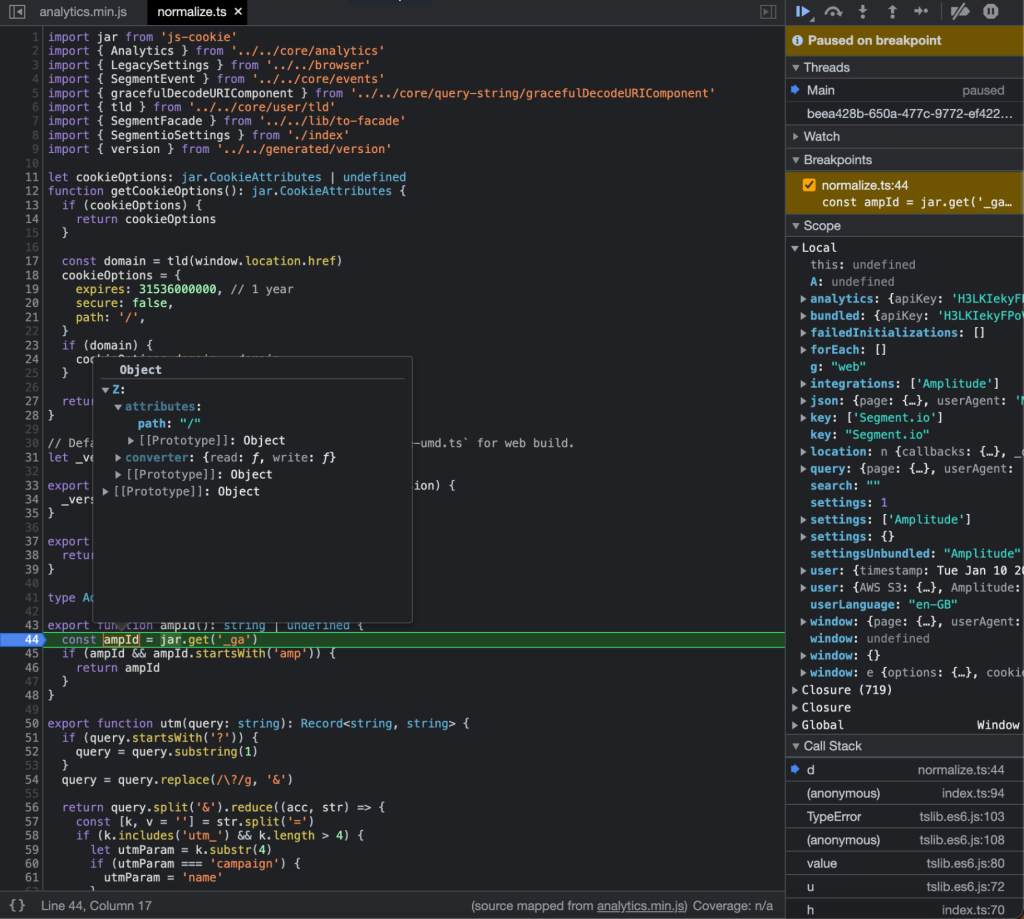
3. Generate a Lighthouse report
Sticking to devtools, did you know you could generate your page report from one of the tabs? If you are using chrome, find your way to the lighthouse tab (which could be utilized as an extension or standalone tool) and let it provide you with a quality report. High chances are at least one part of your page needs improvement.
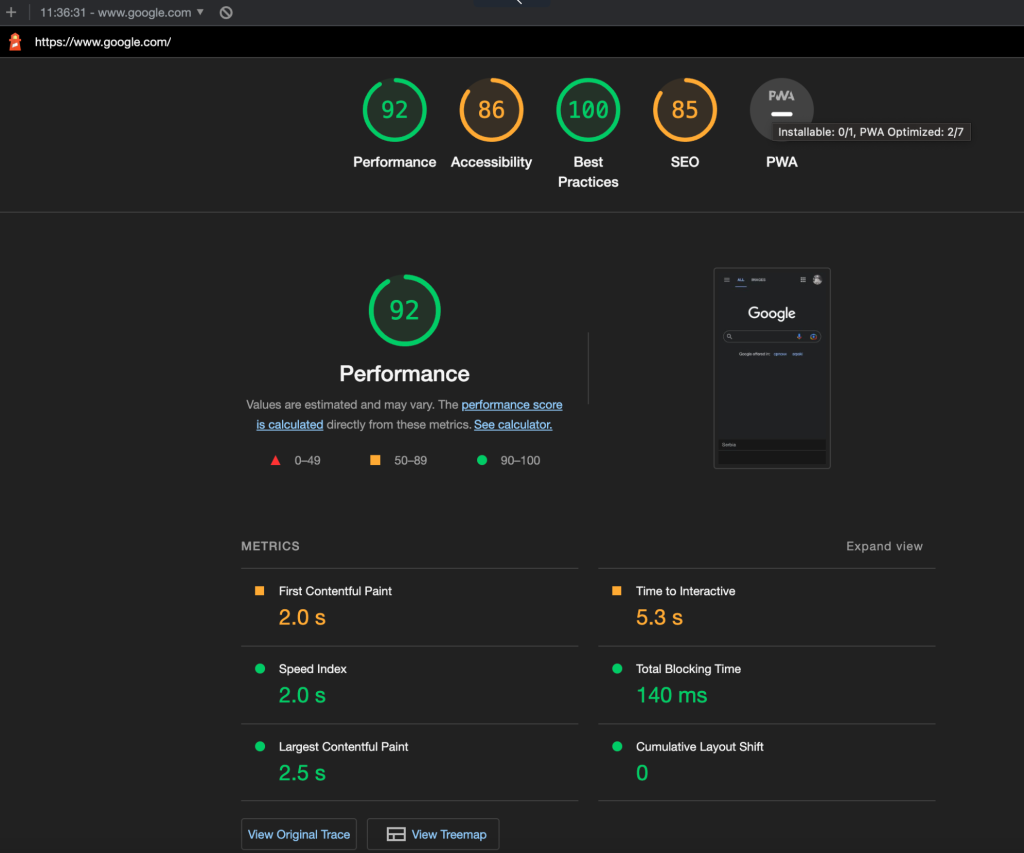
4. Convert Your JSON to Typescript Interfaces
Ever used typescript and had a thought like, “Damn, wouldn’t it be nice if only I could convert this JSON response to a typescript interface?”. Well, actually, there is an extension that could provide you with this functionality if you are using Visual Studio Code as your text editor. We will look at one of many – and that one is JSON to JS by author MariusAlchimavicius.
After adding the extension, highlight your JSON selection, press CMD + SHIFT + P / CTRL + SHIFT + P, and simply search for “JSON to TS: Convert from Selection,” and voila! – your interfaces are ready like below.
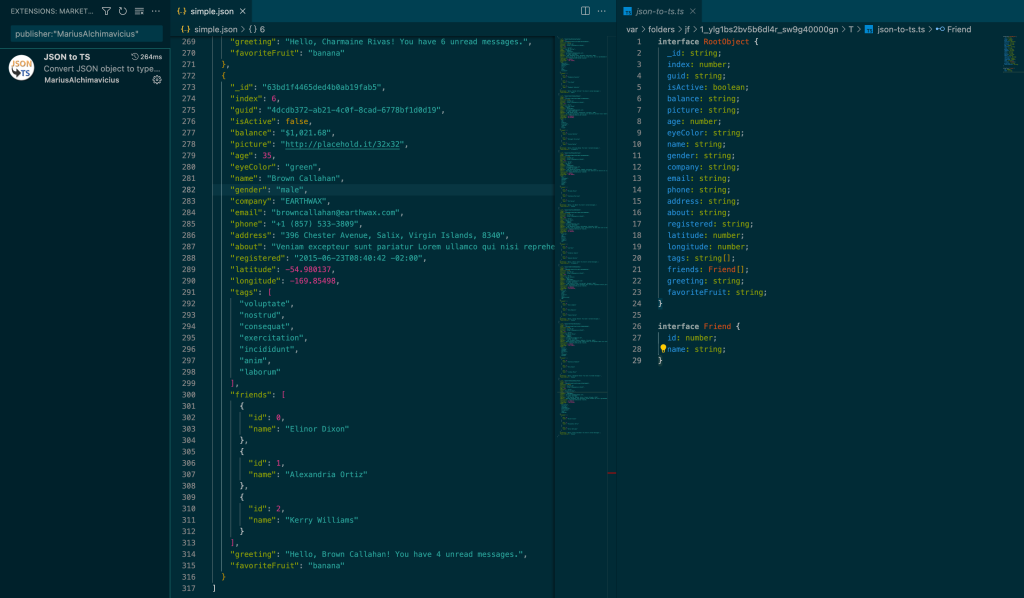
5. Use Inset CSS Property
div {
position: absolute;
top: 0;
left: 0;
bottom: 0;
right: 0;
}
Well, try this next time. Much cleaner
div {
position: absolute;
inset: 0;
}
6. Git Your Way to Glory
Let’s switch to git tricks but stay in the simple universe. Ever wanted to do some git housekeeping? Well, it turns out you could delete all your local branches that don’t have an origin partner by simply running
git remote prune origin
Neat, right?
7. Oh Sh**t, Git!
This one is still related to git, but more in general. Chances are, if you run into a git problem, https://ohshitgit.com/ has your back. Pardon their language, but they have created a so-called library of most common git-related issues, and your today’s pain might find its resolution via a couple of mouse wheel scrolls down. Feel free to check it out – a fun and accessible resource.
Bonus: And a funny one 🙂
Disclaimer, this one is just for laughs and to end the post playfully 🙂
Last but not least – ever felt like your code repo could benefit from narrowing down on size? Look no further than https://thanosjs.org/, a massive improvement in removing a ton of (probably?) unnecessary files.
Thanks for reading the whole thing, and make sure to be on the lookout for more parts of this series. Catch you later!
About Author
Brane Opacic is a well-rounded, dynamic frontend engineer and rising star in the field. With a remarkable 5+ -year career, Brane has established himself as a leading expert in JavaScript, specializing in delivering high-profile solutions for top clients in the Retail, Real-estate, Entertainment, and Sports industries. Brane’s expertise in the field has helped boost the efficiency of deskless workers, streamlined venue-staff management for the leading Europe Football Association, and provided essential tools for Dutch real-estate agencies.